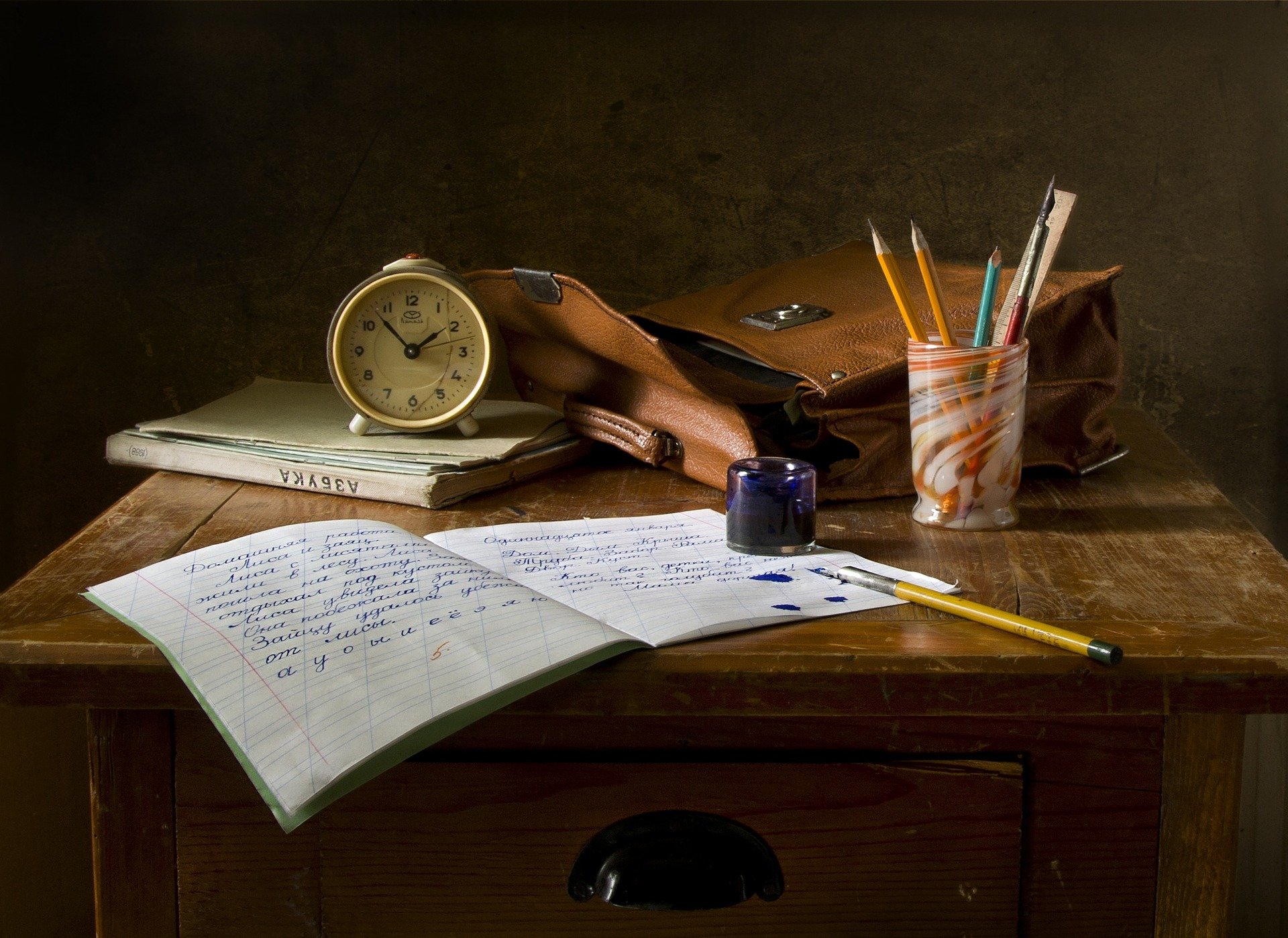
braket rotating
Lv2
wordsCount: 473
readingTime: 3 mins
viewers:
Problem
Define a string as a “valid bracket string” if it follows the given rules:
- “()”, “[]”, and “{}” are all valid bracket strings.
- If
A
is a valid bracket string, then “(A)”, “[A]”, and “{A}” are also valid bracket strings. For example, since “[]” is a valid bracket string, “([])” is also valid. - If
A
andB
are valid bracket strings, then the concatenation “AB” is also a valid bracket string. For example, “{}” and “([])” are valid, so “{}([])” is valid as well.
Given a string s
composed of brackets, when the string is rotated left by x
positions (0 ≤ x < length of s
), determine how many rotations make s
a valid bracket string. Return this count.
Constraints
- The length of
s
is between 1 and 1,000.
Example
s | result |
---|---|
“{}” | 3 |
“}]()[{” | 2 |
“[)(]” | 0 |
“}}}” | 0 |
Explanation of Examples
-
- Rotating the string in various ways results in different strings. Of these rotations, 3 of them result in a valid bracket string.
-
Example 2: “}]()[{”
- For this string, rotating it produces various configurations. Only 2 rotations result in a valid bracket string.
-
Example 3: “[)(]”
- No rotation of this string results in a valid bracket string.
-
Example 4: “}}}
- No rotation of this string results in a valid bracket string.
Each string needs to be checked for validity after each possible rotation to determine if it becomes a valid bracket string. The function should count how many of these rotated versions are valid and return that count.
How to Approach?
Code
|
|
Table of Contents
Related Posts
tuple
Problem A “tuple” is defined as an ordered collection of elements which can include duplicate values and each element is assigned an order. A tuple with n elements (a1, a2, a3, ..., an) can be expressed using the set notation in various ways, with any ordering of the subsets as:
2024-5-1
Tournament
Problem In the Jay’s game tournament, N players compete in a knockout format. Each player is sequentially assigned a number from 1 to N. The matches are arranged as 1 vs 2, 3 vs 4, …, N-1 vs N. The winner of each game advances to the next round, where they receive new sequential numbers starting from 1 for the advancing players.
2024-4-25
Last and First
Problem Several people are playing the game of English word chain where each player takes turns saying a word that must begin with the last letter of the previous word. Here are the rules:
Players take turns in a sequence from 1 to n. After the last player, it starts again with the first player.
2024-4-23
High Score Kit: Stack&Queue#2
Problem The Programmers team is currently performing feature improvement tasks. Each feature can only be released when its progress reaches 100%. However, since the development speed of each feature varies, it is possible for features developed later to be completed earlier. In such cases, a feature that is developed later will be released together with the feature in front of it that is scheduled for earlier release.
2024-4-10
High Score Kit: Stack&Queue#1
Problem Given an array arr consisting of integers ranging from 0 to 9, the task is to remove consecutive duplicates while preserving the order of the remaining elements. For instance, if arr = [1, 1, 3, 3, 0, 1, 1], the result should be [1, 3, 0, 1]. Similarly, for arr = [4, 4, 4, 3, 3], the result should be [4, 3].
2024-4-10
High Score Kit: Greedy#1
Problem Given a number, your task is to remove k digits from the number in such a way that the remaining digits form the largest possible number. The number is provided as a string number and the integer k represents the number of digits to remove. Your function solution should return the resulting largest number as a string.
2024-4-11
Sponsor
Wechat
Alipay
